PHP:
:DrawWeaponIcon
/*
by AJOM
Usage:
0AB1: @DrawWeaponIcon 9 _WeaponID 0@ _atGameScreenCoordinates_XY 1@ 2@ _withSize_WH 3@ 4@ _Color_RGBA 5@ 6@ 7@ 8@
Notes:
- Uses the Rendering region's(0xA94B68) array from 0 to 45 ; RwTexture[0] to RwTexture[45]. Avoid using those regions for example:
* 038D: draw_texture 1 position 1@ 2@ size 3@ 4@ RGBA 5@ 6@ 7@ 8@ // texture index of 1 means RwTexture[0] that is used by the FIST Icon. If you used this elsewhere then the FIST Icon will not be drawn by this snippet
* 038D: draw_texture 35 position 1@ 2@ size 3@ 4@ RGBA 5@ 6@ 7@ 8@ // texture index of 35 means RwTexture[34] that is used by the SNIPER Icon. If you used this elsewhere then the SNIPER Icon will not be drawn by this snippet
* 038D: draw_texture 47 position 1@ 2@ size 3@ 4@ RGBA 5@ 6@ 7@ 8@ // texture index of 47 means RwTexture[46] that is used by the PARACHUTE Icon. If you used this elsewhere then the SNIPER Icon will not be drawn by this snippet
* 038D: draw_texture 48 position 1@ 2@ size 3@ 4@ RGBA 5@ 6@ 7@ 8@ // index from 48 and above can be used, since it's not used by this snippet.
- Uses Game Screen Coords(640x448 Resolution). Keep that in mind that Screen coords is different from game screen coords.
- The weapon needs to be loaded first. Like the weapon was equipped by any actor ingame. The icon will not show if it's not loaded
*/
if 0AB1: @ConvertWeaponIDintoTXDInfo 1 _WeaponID 0@ _Results: _TXDDictionaryName 31@ _TextureName 30@
then
if 0AB1: @FindNamedTextureFromTXDDictionary 2 _TXDDictionaryName 31@ _TextureName 30@ _StoreTexturePointer 31@
then
0A90: 30@ = 0@ * 4
30@ += 0xA94B68 // RwTexture::WeaponID
0A8C: write_memory 30@ size 4 value 31@ virtual_protect 0
0@++ // start from offset 1
03F0: enable_text_draw 1
038D: draw_texture 0@ position 1@ 2@ size 3@ 4@ RGBA 5@ 6@ 7@ 8@
end
end
0AB2: cleo_return 0
:FindNamedTextureFromTXDDictionary
0AA7: | _CTexDictionary__txdIndexByName 0x731850 1 1 | _TXDDictionaryName 0@ _StoreIndex 31@
if 31@ <> -1
then
0AB1: @txdIndexToRwTexDictionary 1 31@ 30@
if 30@ <> 0
then 0AA7: | _RwTexDictionaryFindNamedTexture 0x7F39F0 2 2 | _TextureName 1@ _TXDDictionary 30@ _StoreTexturePointer 29@
end
end
if 29@ > 0
then 0485: return_true
else 059A: return_false
end
0AB2: ret 1 29@
:txdIndexToRwTexDictionary
0A8D: 1@ = read_memory 0xC8800C size 4 virtual_protect 0
0A8E: 2@ = 1@ + 4
0A8D: 2@ = read_memory 2@ size 4 virtual_protect 0
005A: 2@ += 0@
0A8D: 2@ = read_memory 2@ size 1 virtual_protect 0
if 2@ > 0
then
0A8D: 1@ = read_memory 1@ size 4 virtual_protect 0
0@ *= 12
005A: 1@ += 0@
0A8D: 1@ = read_memory 1@ size 4 virtual_protect 0
else 1@ = 0
end
0AB2: ret 1 1@
:ConvertWeaponIDintoTXDInfo
0AC6: 31@ = label @TXDDictionaryName pointer
0AC6: 30@ = label @TextureName pointer
if 0@ == 0
then
0AD3: 31@ = string_format "hud"
0AD3: 30@ = string_format "fist"
else if 0@ == 1
then
0AD3: 31@ = string_format "brassknuckle"
0AD3: 30@ = string_format "BRASSKNUCKLEicon"
else if 0@ == 2
then
0AD3: 31@ = string_format "golfclub"
0AD3: 30@ = string_format "golfclubicon"
else if 0@ == 3
then
0AD3: 31@ = string_format "nitestick"
0AD3: 30@ = string_format "nitestickicon"
else if 0@ == 4
then
0AD3: 31@ = string_format "knifecur"
0AD3: 30@ = string_format "knifecuricon"
else if 0@ == 5
then
0AD3: 31@ = string_format "bat"
0AD3: 30@ = string_format "baticon"
else if 0@ == 6
then
0AD3: 31@ = string_format "shovel"
0AD3: 30@ = string_format "shovelicon"
else if 0@ == 7
then
0AD3: 31@ = string_format "poolcue"
0AD3: 30@ = string_format "poolcueicon"
else if 0@ == 8
then
0AD3: 31@ = string_format "katana"
0AD3: 30@ = string_format "katanaicon"
else if 0@ == 9
then
0AD3: 31@ = string_format "chnsaw"
0AD3: 30@ = string_format "chnsawicon"
else if 0@ == 10
then
0AD3: 31@ = string_format "gun_dildo1"
0AD3: 30@ = string_format "gun_dildo1icon"
else if 0@ == 11
then
0AD3: 31@ = string_format "gun_dildo2"
0AD3: 30@ = string_format "gun_dildo2icon"
else if 0@ == 12
then
0AD3: 31@ = string_format "gun_vibe1"
0AD3: 30@ = string_format "gun_vibe1icon"
else if 0@ == 13
then
0AD3: 31@ = string_format "gun_vibe2"
0AD3: 30@ = string_format "gun_vibe2icon"
else if 0@ == 14
then
0AD3: 31@ = string_format "flowera"
0AD3: 30@ = string_format "floweraicon"
else if 0@ == 15
then
0AD3: 31@ = string_format "gun_cane"
0AD3: 30@ = string_format "gun_caneicon"
else if 0@ == 16
then
0AD3: 31@ = string_format "grenade"
0AD3: 30@ = string_format "grenadeicon"
else if 0@ == 17
then
0AD3: 31@ = string_format "teargas"
0AD3: 30@ = string_format "teargasicon"
else if 0@ == 18
then
0AD3: 31@ = string_format "molotov"
0AD3: 30@ = string_format "molotovicon"
else if 0@ == 22
then
0AD3: 31@ = string_format "colt45"
0AD3: 30@ = string_format "colt45icon"
else if 0@ == 23
then
0AD3: 31@ = string_format "silenced"
0AD3: 30@ = string_format "silencedicon"
else if 0@ == 24
then
0AD3: 31@ = string_format "desert_eagle"
0AD3: 30@ = string_format "desert_eagleicon"
else if 0@ == 25
then
0AD3: 31@ = string_format "chromegun"
0AD3: 30@ = string_format "chromegunicon"
else if 0@ == 26
then
0AD3: 31@ = string_format "sawnoff"
0AD3: 30@ = string_format "sawnofficon"
else if 0@ == 27
then
0AD3: 31@ = string_format "shotgspa"
0AD3: 30@ = string_format "shotgspaicon"
else if 0@ == 28
then
0AD3: 31@ = string_format "micro_uzi"
0AD3: 30@ = string_format "micro_uziicon"
else if 0@ == 29
then
0AD3: 31@ = string_format "mp5lng"
0AD3: 30@ = string_format "mp5lngicon"
else if 0@ == 30
then
0AD3: 31@ = string_format "ak47"
0AD3: 30@ = string_format "ak47icon"
else gosub @weapontxdcondition
end
end
end
end
end
end
end
end
end
end
end
end
end
end
end
end
end
end
end
end
end
end
end
end
end
end
end
end
if or
31@ == 0
30@ == 0
then 059A: return_false
else 0485: return_true
end
ret 2 31@ 30@
:weapontxdcondition
if 0@ == 31
then
0AD3: 31@ = string_format "m4"
0AD3: 30@ = string_format "m4icon"
else if 0@ == 32
then
0AD3: 31@ = string_format "tec9"
0AD3: 30@ = string_format "tec9icon"
else if 0@ == 33
then
0AD3: 31@ = string_format "cuntgun"
0AD3: 30@ = string_format "cuntgunicon"
else if 0@ == 34
then
0AD3: 31@ = string_format "sniper"
0AD3: 30@ = string_format "snipericon"
else if 0@ == 35
then
0AD3: 31@ = string_format "rocketla"
0AD3: 30@ = string_format "rocketlaicon"
else if 0@ == 36
then
0AD3: 31@ = string_format "heatseek"
0AD3: 30@ = string_format "heatseekicon"
else if 0@ == 37
then
0AD3: 31@ = string_format "flame"
0AD3: 30@ = string_format "flameicon"
else if 0@ == 38
then
0AD3: 31@ = string_format "minigun"
0AD3: 30@ = string_format "minigunicon"
else if 0@ == 39
then
0AD3: 31@ = string_format "satchel"
0AD3: 30@ = string_format "satchelicon"
else if 0@ == 40
then
0AD3: 31@ = string_format "bomb"
0AD3: 30@ = string_format "bombicon"
else if 0@ == 41
then
0AD3: 31@ = string_format "spraycan"
0AD3: 30@ = string_format "spraycanicon"
else if 0@ == 42
then
0AD3: 31@ = string_format "fire_ex"
0AD3: 30@ = string_format "fire_exicon"
else if 0@ == 43
then
0AD3: 31@ = string_format "camera"
0AD3: 30@ = string_format "cameraicon"
else if 0@ == 44
then
0AD3: 31@ = string_format "nvgoggles"
0AD3: 30@ = string_format "nvgogglesicon"
else if 0@ == 45
then
0AD3: 31@ = string_format "irgoggles"
0AD3: 30@ = string_format "irgogglesicon"
else if 0@ == 46
then
0AD3: 31@ = string_format "gun_para"
0AD3: 30@ = string_format "gun_paraicon"
else
31@ = 0
30@ = 0
end
end
end
end
end
end
end
end
end
end
end
end
end
end
end
end
return
:TXDDictionaryName
hex
00 00 00 00 00
00 00 00 00 00
00 00 00
end
:TextureName
hex
00 00 00 00 00
00 00 00 00 00
00 00 00 00 00
00 00
end
Code:
{$CLEO}
0000:
while true
wait 0
// The weapon needs to be loaded first. Like the weapon was equipped by any actor ingame. The icon will not show if it's not loaded
0AB1: @DrawWeaponIcon 9 _WeaponID 24 _atGameScreenCoordinates_XY 160.0 112.0 _withSize_WH 50.0 50.0 _Color_RGBA 255 255 255 255 // deagle icon
0AB1: @DrawWeaponIcon 9 _WeaponID 31 _atGameScreenCoordinates_XY 160.0 336.0 _withSize_WH 40.0 40.0 _Color_RGBA 255 255 255 255 // m4 icon
0AB1: @DrawWeaponIcon 9 _WeaponID 38 _atGameScreenCoordinates_XY 480.0 112.0 _withSize_WH 30.0 30.0 _Color_RGBA 255 255 255 255 // minigun icon
0AB1: @DrawWeaponIcon 9 _WeaponID 9 _atGameScreenCoordinates_XY 480.0 336.0 _withSize_WH 20.0 20.0 _Color_RGBA 255 255 255 255 // chainsaw icon
end
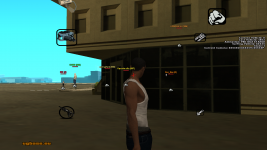